Python programming is a versatile and powerful tool that allows developers to create efficient and effective solutions for a wide range of applications. One of the key aspects of Python is its ability to iterate through counters, which is an essential function in many programming tasks. By mastering the technique of iterating through a counter in Python, programmers can optimize their code, enhance its functionality, and streamline complex processes.
In this article, we will delve into the intricacies of iterating through a counter in Python, exploring its importance, various methods, and practical applications. We'll cover everything from the basics of counters to more advanced techniques, ensuring that you gain a comprehensive understanding of how to utilize this feature in your projects. Whether you're a beginner looking to expand your Python skills or an experienced developer seeking to refine your coding practices, this guide will provide valuable insights and tips.
By the end of this article, you'll have a thorough grasp of iterating through a counter in Python, enabling you to write more efficient and effective code. We'll also address common questions and challenges that arise when working with counters, providing solutions and best practices to help you overcome any obstacles. So, let's dive in and discover the power of iterating through a counter in Python!
Read also:Charleston White A Dynamic And Controversial Figure In Modern Society
Table of Contents
- What are Counters in Python?
- Why Iterate Through a Counter?
- Basic Iteration Techniques
- Advanced Iteration Methods
- Using Counters with Loops
- How Do You Handle Exceptions?
- How to Optimize Counter Iteration?
- Iterating Through a Counter Python in Real-World Applications
- Common Mistakes
- Debugging Counter Issues
- What are Best Practices?
- How Iterating Counters Enhances Performance?
- Tools and Libraries
- FAQs
- Conclusion
What are Counters in Python?
Counters in Python are a part of the collections module, a specialized container type that is optimized for counting elements. Essentially, a counter is a dictionary subclass that helps count hashable objects. It is extremely useful in situations where you want to keep track of the frequency of elements in an iterable or perform operations based on these frequencies.
Counters can be created using the Counter()
class from the collections module. They allow for various operations such as addition, subtraction, and intersection, providing an easy way to manipulate and analyze data. Here’s a basic example of a counter:
from collections import Counter # Create a counter from a list counter = Counter(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']) # Display the counter print(counter) # Output: Counter({'banana': 3, 'apple': 2, 'orange': 1})
This example demonstrates how a counter can be used to count the occurrences of each item in a list. The counter object stores elements as dictionary keys and their counts as dictionary values.
Why Iterate Through a Counter?
Iterating through a counter is crucial in programming as it allows developers to perform operations on each element based on their frequency. This can be particularly useful in tasks such as data analysis, statistical computation, and machine learning, where understanding the distribution of elements is key to making informed decisions.
By iterating through a counter, you can efficiently:
- Analyze data patterns and trends.
- Filter and sort data based on frequency.
- Perform aggregate operations like summation and averaging.
- Debug and optimize code by understanding data distribution.
In addition, counters provide a convenient way to deal with large datasets, as they offer a memory-efficient way to store and process counts. This makes iterating through a counter an essential skill for any Python programmer who deals with data-intensive applications.
Read also:Inspirational Insights Rainbow Quote And Its Vibrant Significance
Basic Iteration Techniques
Understanding the basic techniques for iterating through a counter is the first step towards harnessing its full potential. The most straightforward approach is to use a for
loop, which allows you to iterate over the elements and their respective counts.
Here’s a simple example:
from collections import Counter # Create a counter counter = Counter(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']) # Iterate through the counter for item, count in counter.items(): print(f"{item}: {count}")
In this example, the items()
method returns each element and its count as key-value pairs, which can be accessed inside the loop. This technique is ideal for situations where you need to perform operations on each element individually.
Another basic technique is the use of list comprehensions, which provide a concise and efficient way to iterate through counters and apply transformations:
# Use a list comprehension to create a list of items with counts greater than 1 filtered_items = [item for item, count in counter.items() if count > 1] print(filtered_items) # Output: ['apple', 'banana']
List comprehensions are a powerful tool in Python, allowing you to filter and transform data in a single line of code. When used with counters, they enable quick and efficient data manipulation.
Advanced Iteration Methods
Once you’ve mastered the basics of iterating through a counter, you can explore more advanced methods to enhance your code’s functionality. One such method is the use of the most_common()
function, which returns the n most common elements and their counts.
Here’s an example:
from collections import Counter # Create a counter counter = Counter(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']) # Get the 2 most common elements most_common_elements = counter.most_common(2) print(most_common_elements) # Output: [('banana', 3), ('apple', 2)]
The most_common()
function is particularly useful in applications where you need to identify the most frequently occurring elements in a dataset, such as in recommendation systems or trend analysis.
Another advanced technique involves combining counters using arithmetic operations. This allows you to perform operations like addition, subtraction, intersection, and union on multiple counters:
# Create two counters counter1 = Counter(['apple', 'banana', 'apple']) counter2 = Counter(['banana', 'orange', 'banana']) # Add the counters combined_counter = counter1 + counter2 print(combined_counter) # Output: Counter({'banana': 3, 'apple': 2, 'orange': 1})
By leveraging these advanced methods, you can build more complex and robust applications that can handle a wide range of data processing tasks.
Using Counters with Loops
Loops play a vital role in iterating through counters, allowing you to process elements based on their frequency. The most common loop used with counters is the for
loop, which enables easy access to each element and its count.
Here’s how you can use a for
loop with a counter:
from collections import Counter # Create a counter counter = Counter(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']) # Use a for loop to iterate through the counter for fruit, count in counter.items(): print(f"The fruit {fruit} appears {count} times.")
In this example, the items()
method provides a convenient way to access each element and its count, allowing you to perform operations on them within the loop.
Another loop that can be used with counters is the while
loop, which is useful in situations where you need to perform actions based on a condition:
# Initialize a counter counter = Counter(['apple', 'banana', 'apple']) # Initialize a variable to store the total count total_count = 0 # Use a while loop to sum the counts while total_count
In this example, the while
loop continues to iterate until the total count reaches a specified value, demonstrating how counters can be used to control loop execution.
How Do You Handle Exceptions?
Handling exceptions is an important aspect of working with counters in Python, as it ensures that your code can gracefully handle unexpected situations. The most common way to handle exceptions is by using try-except blocks, which allow you to catch and respond to errors.
Here’s an example of how to handle exceptions when iterating through a counter:
from collections import Counter # Create a counter counter = Counter(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']) # Use a try-except block to handle exceptions try: # Attempt to access a non-existent key count = counter['pear'] except KeyError: print("The key 'pear' does not exist in the counter.")
In this example, the try block attempts to access a key that does not exist in the counter, and the except block catches the resulting KeyError, providing a user-friendly message. This approach ensures that your code remains robust and can handle unexpected inputs.
Another common exception that may arise when working with counters is the TypeError, which occurs when attempting to perform an operation on incompatible data types. To handle this, you can use additional except clauses:
try: # Attempt to add a counter to a non-counter object result = counter + 5 except TypeError: print("Cannot add a counter to a non-counter object.")
By handling exceptions effectively, you can ensure that your code remains reliable and user-friendly, even in the face of unexpected inputs or errors.
How to Optimize Counter Iteration?
Optimizing counter iteration is essential for improving the performance and efficiency of your code. Here are some techniques to consider:
- Use Built-In Functions: Python's built-in functions, such as
sum()
,min()
, andmax()
, can be used to perform operations on counters efficiently. These functions are implemented in C and are often faster than equivalent Python code. - Avoid Unnecessary Computations: When iterating through a counter, avoid performing redundant calculations. For instance, if you've already computed the total count of elements, store it in a variable rather than recalculating it each time.
- Leverage List Comprehensions: List comprehensions provide a concise and efficient way to filter and transform data in a single line. By using list comprehensions, you can reduce the number of lines of code and improve readability.
- Utilize Generators: Generators offer a memory-efficient way to iterate through large datasets. By using a generator expression instead of a list comprehension, you can iterate over elements one at a time, reducing memory usage.
By implementing these optimization techniques, you can enhance the performance of your code and ensure that it runs efficiently, even when processing large datasets.
Iterating Through a Counter Python in Real-World Applications
Iterating through a counter in Python is a valuable skill that can be applied to a wide range of real-world applications. Here are some examples of where this technique can be used effectively:
- Data Analysis: Counters are often used in data analysis to count occurrences of categories in datasets, such as counting the number of times each word appears in a text document or the frequency of different events in a log file.
- Natural Language Processing (NLP): In NLP, counters are used to create word frequency distributions, which are essential for tasks like text classification, sentiment analysis, and language modeling.
- Machine Learning: Counters are used to preprocess data and create features for machine learning models. For instance, they can be used to count the number of occurrences of different labels in a dataset.
- E-Commerce: In e-commerce applications, counters can be used to track product sales, customer interactions, and other metrics, helping businesses make data-driven decisions.
By mastering the technique of iterating through a counter in Python, you can unlock new possibilities and applications for your projects, enabling you to tackle complex data processing tasks with ease.
Common Mistakes
Despite its power and versatility, working with counters in Python can lead to common mistakes that may affect the functionality and performance of your code. Here are some pitfalls to watch out for:
- Ignoring Data Types: Counters only work with hashable objects, so attempting to use unhashable types, such as lists, as keys will result in a TypeError. Ensure that the data you’re working with is compatible with counters.
- Overlooking KeyError Exceptions: Accessing a key that does not exist in a counter will raise a KeyError. To avoid this, use the
get()
method, which returns a default value if the key is not present. - Using Counters for Mutable Operations: Counters are designed for counting immutable elements. If you need to perform operations that modify elements, consider using other data structures, such as lists or dictionaries.
- Not Leveraging Built-In Functions: Python provides a range of built-in functions and methods that can simplify and optimize operations on counters. Familiarize yourself with these functions to enhance your code’s efficiency.
By being aware of these common mistakes and taking steps to avoid them, you can ensure that your code remains robust, efficient, and free of errors.
Debugging Counter Issues
Debugging issues with counters can be challenging, but with the right approach, you can identify and resolve problems effectively. Here are some strategies for debugging counter-related issues:
- Use Print Statements: Print statements are a simple yet effective way to understand the state of your counter at different points in your code. Use them to print the contents of your counter and check for discrepancies.
- Check Data Types: Ensure that the data types of elements in your counter are consistent. Inconsistent data types can lead to unexpected behavior and errors.
- Verify Key Existence: Before accessing a key in a counter, verify its existence using the
in
keyword. This can prevent KeyError exceptions and help you identify missing keys. - Use Python’s Debugger: Python’s built-in debugger,
pdb
, allows you to step through your code and examine variables at each step. This can be especially helpful for identifying issues with counter operations.
By employing these debugging techniques, you can effectively troubleshoot and resolve issues related to counters, ensuring that your code runs smoothly and efficiently.
What are Best Practices?
To make the most of iterating through a counter in Python, it’s important to follow best practices that ensure your code is efficient, readable, and maintainable. Here are some best practices to consider:
- Use Descriptive Variable Names: Choose variable names that clearly describe the purpose of the counter and the data it contains. This improves code readability and makes it easier to understand.
- Leverage Built-In Methods: Python provides a range of built-in methods, such as
most_common()
andelements()
, that can simplify and optimize counter operations. Familiarize yourself with these methods and use them when appropriate. - Document Your Code: Include comments and documentation to explain the purpose and functionality of your code. This is especially important when working with complex counters or performing advanced operations.
- Test Your Code: Regularly test your code to ensure that it behaves as expected. Use test cases to verify the correctness of counter operations and handle edge cases effectively.
By adhering to these best practices, you can write high-quality Python code that effectively utilizes counters and delivers optimal performance.
How Iterating Counters Enhances Performance?
Iterating through counters can significantly enhance the performance of your Python code by providing an efficient way to process and analyze data. Here’s how counters contribute to improved performance:
- Memory Efficiency: Counters are implemented as dictionary subclasses, which offer a memory-efficient way to store and process counts. This allows you to work with large datasets without consuming excessive memory.
- Fast Access and Retrieval: Counters provide fast access to elements and their counts, enabling quick retrieval and processing. This is particularly beneficial in applications where performance is critical.
- Optimized Operations: Counters come with built-in methods for common operations, such as finding the most common elements or adding multiple counters. These methods are optimized for performance, allowing you to perform complex tasks efficiently.
- Scalability: Counters can handle large volumes of data and scale well with increasing dataset sizes. This makes them suitable for applications that require processing of extensive data.
By leveraging the performance benefits of counters, you can build applications that are both efficient and capable of handling complex data processing tasks.
Tools and Libraries
Python offers a wealth of tools and libraries that can enhance your ability to work with counters and perform data analysis tasks. Here are some popular tools and libraries to consider:
- Pandas: Pandas is a powerful data analysis library that provides data structures and functions for working with structured data. It offers DataFrames, which are ideal for storing and analyzing datasets.
- NumPy: NumPy is a fundamental package for scientific computing in Python. It provides support for arrays and matrices, along with a range of mathematical functions for performing operations on these data structures.
- Matplotlib: Matplotlib is a plotting library that allows you to create visualizations of your data. It is particularly useful for creating charts, graphs, and other visual representations of counter data.
- Scikit-learn: Scikit-learn is a machine learning library that provides tools for data mining and data analysis. It includes built-in functions for preprocessing data and creating machine learning models.
By incorporating these tools and libraries into your projects, you can enhance your ability to work with counters and perform advanced data analysis tasks effectively.
FAQs
Here are some frequently asked questions about iterating through a counter in Python:
- Can counters be used with non-numeric data? Yes, counters can be used with any hashable data type, including strings, tuples, and other immutable types. This makes them versatile for counting occurrences of various elements.
- How do I reset a counter? You can reset a counter by assigning it an empty counter, such as
Counter()
, or by using theclear()
method to remove all elements. - Can counters handle negative counts? Yes, counters can handle negative counts, which can be useful in certain applications, such as tracking resource usage or performing arithmetic operations between counters.
- Is it possible to sort a counter? While counters themselves do not maintain order, you can sort them by converting them to a list of tuples and using Python’s built-in
sorted()
function. - How do I find the least common elements in a counter? To find the least common elements, you can use the
most_common()
method with a negative argument or sort the counter and retrieve the desired elements. - Are counters thread-safe? No, counters are not inherently thread-safe. If you need to use counters in a multithreaded environment, consider using locks or other synchronization mechanisms to ensure thread safety.
Conclusion
Iterating through a counter in Python is a powerful technique that can enhance your ability to process and analyze data efficiently. By mastering this skill, you can unlock new possibilities for your projects, enabling you to tackle complex data processing tasks with ease. Whether you’re working in data analysis, machine learning, or any other field that involves data manipulation, understanding how to iterate through a counter is an essential tool in your Python programming arsenal.
By following best practices, optimizing your code, and leveraging the tools and libraries available in Python, you can ensure that your applications are efficient, robust, and capable of handling even the most challenging data processing tasks. As you continue to explore the capabilities of iterating through a counter in Python, you’ll discover new ways to improve your code’s functionality and performance, enabling you to create more effective and impactful solutions.
Remember to experiment with different techniques, stay updated with the latest developments in Python, and continuously refine your skills. With dedication and practice, you’ll become proficient in iterating through counters and harness the full potential of Python programming.
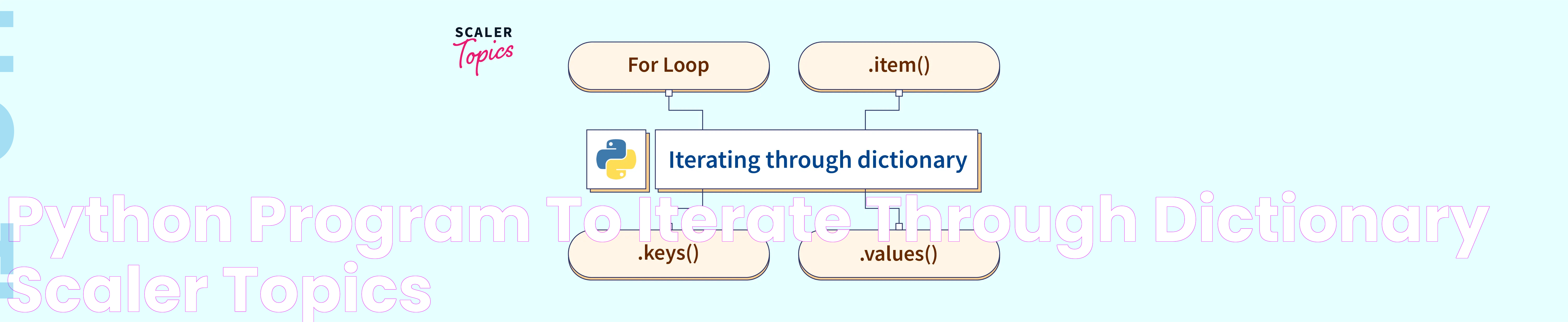
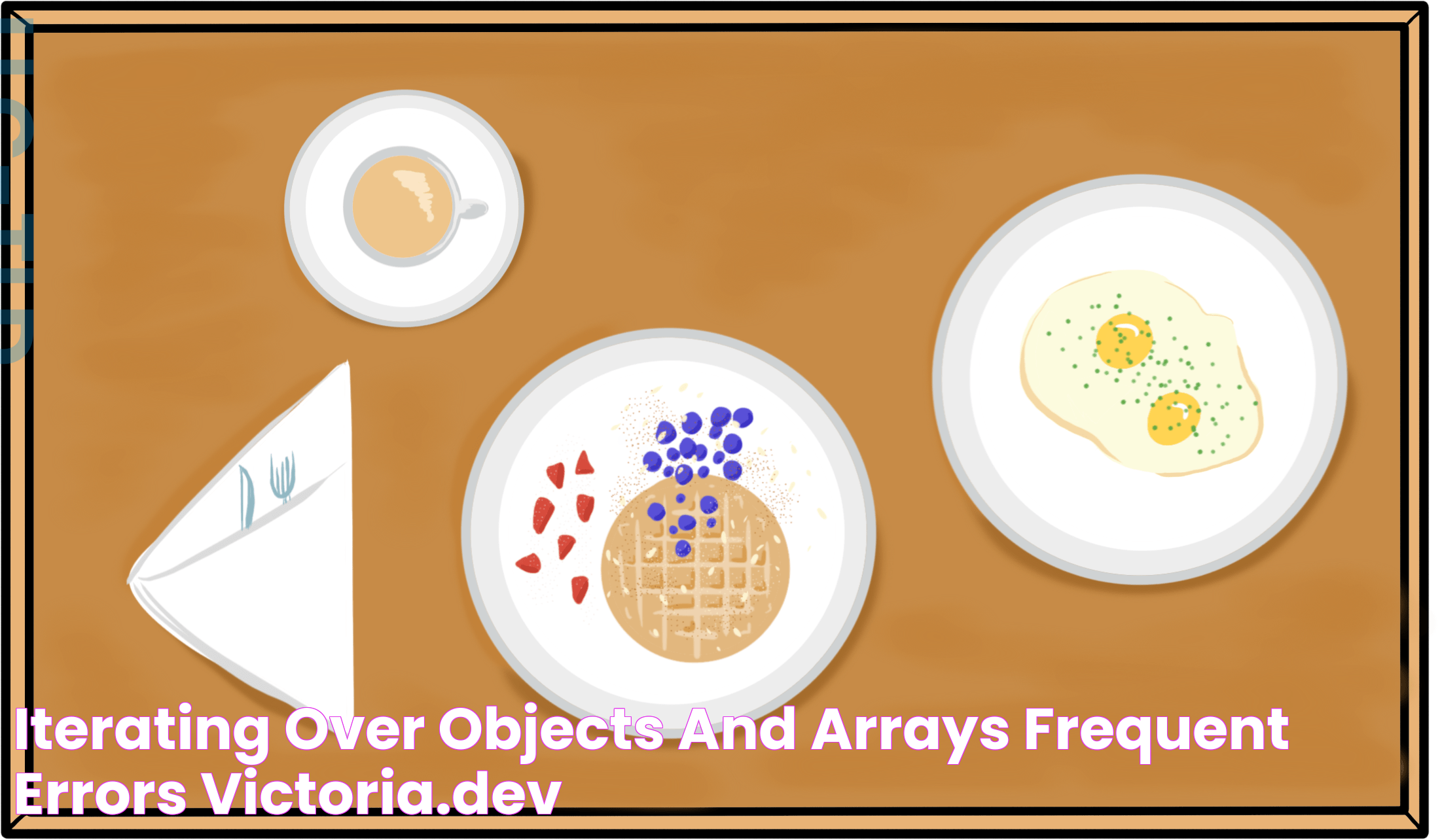