JavaScript is a powerful language that provides developers with a wide range of tools to manipulate and work with data. One such tool is the "foreach" method, which allows developers to efficiently iterate over arrays, applying a specified function to each element. This method is particularly useful for tasks that require processing or transforming data, making it a staple in any developer's toolkit. For those who are new to programming or JavaScript, understanding how to use the "foreach" method can greatly enhance your ability to work with arrays and data structures. Whether you're building web applications, data analysis tools, or any other type of software, mastering this method is essential.
In this article, we will explore the various aspects of the "javascript foreach" method, including its syntax, use cases, and best practices. We will also compare it to other iteration methods available in JavaScript, such as "for", "for...in", and "for...of", to provide a comprehensive understanding of when and why to use "foreach". By the end of this article, you should have a solid grasp of how to effectively utilize this method in your projects.
Moreover, we aim to provide a detailed, SEO-friendly, and reader-engaging guide that adheres to Google Discover's rules. This means our content is not only informative but also structured in a way that ensures it is easily indexed and potentially featured in Google's Discover feed. So, let's embark on this journey to enhance your coding skills with "javascript foreach".
Read also:Benefits Of Electron Microscopes A Deep Dive Into The Microscopic World
Table of Contents
- What is JavaScript foreach?
- Why Use JavaScript foreach?
- How to Use JavaScript foreach?
- JavaScript foreach vs. For Loop
- JavaScript foreach vs. Map
- Common Errors with JavaScript foreach
- Best Practices for JavaScript foreach
- JavaScript foreach and Asynchronous Operations
- Advanced Uses of JavaScript foreach
- JavaScript foreach in Modern Web Development
- JavaScript foreach in Frameworks and Libraries
- Performance Considerations with JavaScript foreach
- Security Implications of JavaScript foreach
- JavaScript foreach in the Future
- FAQs
- Conclusion
What is JavaScript foreach?
The "javascript foreach" method is an integral part of the JavaScript language, designed specifically for iterating over arrays. It executes a provided function once for each array element, in ascending order, and is considered one of the most straightforward ways to loop through an array without managing the loop's counter variables. This method simplifies code and reduces potential errors associated with traditional loop constructs.
One significant advantage of using "foreach" is its ability to enhance code readability. By abstracting the iteration process, developers can focus on the core logic of their application without getting bogged down by the mechanics of iteration. Additionally, "foreach" is part of the Array.prototype, meaning it is available on all array instances and adheres to the functional programming paradigm prevalent in modern JavaScript.
It's important to note that "foreach" does not execute the function for empty elements. This behavior differentiates it from other iteration methods, such as "map" or "reduce", which apply the function to every index, regardless of whether it is assigned a value. Understanding these nuances is crucial for developers who wish to leverage "foreach" effectively in their code.
Why Use JavaScript foreach?
There are several compelling reasons to use the "javascript foreach" method in your projects. Firstly, it simplifies the process of iterating over arrays by abstracting the loop control structure. This simplicity can lead to cleaner, more maintainable code, which is easier to read and understand, especially in large codebases or team environments where multiple developers are involved.
Another reason to use "foreach" is its alignment with functional programming principles. By treating functions as first-class citizens and passing them as arguments to other functions, developers can create more modular and reusable code. This approach not only fosters code reuse but also encourages the development of smaller, more focused functions that do one thing and do it well.
Moreover, the "foreach" method is inherently safe to use in terms of code execution order. Unlike traditional loops that can be influenced by manual increment or decrement operations, "foreach" guarantees that the provided function will be called once for each element in the array, in the order they appear. This predictability is essential for applications where the sequence of operations matters, such as data processing or transformation tasks.
Read also:Christopher Reeve A Heros Final Days And Lasting Impact
How to Use JavaScript foreach?
Using the "javascript foreach" method is straightforward and involves calling the method on an array and passing a callback function as an argument. This callback function is applied to each element of the array in turn, enabling developers to perform operations such as modifying elements, logging them, or accumulating a value. Here's a basic example:
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
In this example, the "foreach" method iterates over the "numbers" array, logging each number to the console. The method can also accept an optional second argument, which sets the value of "this" within the callback function. This feature can be useful when working with object-oriented code or when a specific execution context is required.
When using "foreach", it's crucial to remember that it does not return a new array. Unlike methods such as "map" or "filter", which generate a new array based on the results of the callback function, "foreach" is intended solely for executing side effects. Therefore, if you need to create a new array based on some transformation, you should consider using "map" instead.
JavaScript foreach vs. For Loop: Which is Better?
The choice between using "javascript foreach" and a traditional "for" loop often depends on the specific requirements of the task at hand. Each method has its advantages and limitations, making them suitable for different scenarios.
Traditional "for" loops offer more control over the iteration process. Developers can manipulate the loop counter, use "break" statements to exit the loop early, and manage the execution flow with greater precision. This flexibility can be beneficial in situations where specific elements need to be skipped, or the loop needs to be terminated based on a condition.
However, this level of control comes at the cost of increased complexity and potential for errors. Manual loop counters and condition checks can lead to off-by-one errors or infinite loops if not correctly managed. In contrast, "foreach" abstracts these concerns, allowing developers to focus solely on the operation being performed on each element, thereby reducing the risk of such mistakes.
In terms of performance, traditional "for" loops can be faster than "foreach" in specific scenarios, particularly when manipulating large datasets or when loop control variables play a significant role. However, the difference is often negligible for most applications, and the benefits of cleaner, more maintainable code often outweigh the slight performance gain.
JavaScript foreach vs. Map: What's the Difference?
Both "javascript foreach" and "map" are methods used to iterate over arrays, but they serve different purposes. Understanding these differences is crucial for selecting the right tool for your task.
The primary distinction lies in their return values. The "foreach" method is designed to execute a function on each element of an array without returning a new array. It is ideal for performing side effects, such as logging or modifying external variables. In contrast, the "map" method returns a new array, with each element being the result of the callback function applied to the original array elements.
Consider the following example, which demonstrates how "map" transforms an array:
const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map(function(number) { return number * number; }); console.log(squaredNumbers); // [1, 4, 9, 16, 25]
In this case, "map" returns a new array containing the squares of the original numbers, making it suitable for tasks that involve transforming data while preserving immutability. If your goal is to create a new array with transformed elements, "map" is the preferred choice.
On the other hand, if your task involves side effects without the need for a new array, "foreach" is the appropriate method. Understanding these differences empowers developers to write more efficient and purpose-driven code.
What Are Common Errors with JavaScript foreach?
Despite its simplicity, developers can encounter several common errors when using the "javascript foreach" method. Being aware of these pitfalls can help avoid potential issues and ensure smooth execution.
- Misunderstanding Return Behavior: One frequent mistake is expecting "foreach" to return a new array. As mentioned earlier, "foreach" does not return any value, which can lead to unexpected results if a developer mistakenly assumes otherwise.
- Incorrect Callback Function: Another common error is passing an incorrect or poorly defined callback function. The function should accept at least one parameter (the current array element) and optionally two more (the index and the array itself). Failing to correctly define these parameters can lead to unintended behavior.
- Using "foreach" on Non-Arrays: Attempting to use "foreach" on non-array objects will result in a TypeError, as "foreach" is specifically designed for array instances. To avoid this, ensure the data structure is an array before calling the method.
By understanding these potential errors and their causes, developers can write more robust and error-free code when working with "foreach".
Best Practices for JavaScript foreach
Adhering to best practices when using "javascript foreach" can help developers write more efficient and maintainable code. Here are some recommendations to consider:
- Use Descriptive Callback Function Names: Naming your callback functions appropriately can enhance code readability and provide context for the operation being performed. Avoid generic names like "callback" or "fn" in favor of descriptive names that convey the function's purpose.
- Keep Functions Concise: Aim to keep the logic within your callback functions concise and focused on a single task. If a function becomes too complex, consider breaking it down into smaller, reusable functions.
- Minimize Side Effects: Avoid unnecessary side effects within your callback functions, as they can lead to unintended consequences. If a side effect is required, document it clearly to ensure other developers understand its purpose.
By following these best practices, developers can harness the full potential of "foreach" while maintaining clean and efficient code.
Can JavaScript foreach Handle Asynchronous Operations?
While "javascript foreach" is primarily designed for synchronous operations, developers can use it in conjunction with asynchronous processes by leveraging promises and async/await syntax. However, it's important to note that "foreach" does not inherently support asynchronous operations, which can lead to unexpected behavior if not handled correctly.
Consider the following example, which demonstrates how to use "foreach" with async/await:
async function processData() { const data = [1, 2, 3, 4, 5]; await Promise.all(data.map(async (item) => { await performAsyncOperation(item); })); } async function performAsyncOperation(item) { return new Promise(resolve => setTimeout(() => { console.log(`Processed item: ${item}`); resolve(); }, 1000)); } processData();
In this example, the "map" method is used instead of "foreach" to ensure that all asynchronous operations are completed before proceeding. The "Promise.all" method waits for all promises to resolve, ensuring that the asynchronous operations are handled correctly.
By understanding how to integrate asynchronous operations with "foreach", developers can effectively manage asynchronous workflows within their applications.
Advanced Uses of JavaScript foreach
The "javascript foreach" method can be applied to a wide range of advanced use cases, enabling developers to create sophisticated applications and solutions. Here are some examples of how "foreach" can be used in more complex scenarios:
- Data Transformation Pipelines: "foreach" can be used to build data transformation pipelines, where each element of an array undergoes a series of transformations. By chaining multiple "foreach" calls, developers can create efficient and modular data processing workflows.
- Custom Iterators: Developers can create custom iterators that leverage "foreach" to iterate over non-standard data structures. By defining custom iterator functions, developers can extend the capabilities of "foreach" to suit their specific needs.
- Parallel Processing: While "foreach" is inherently sequential, developers can implement parallel processing techniques by using "foreach" in conjunction with web workers or other parallel computing strategies. This approach can significantly improve performance for computationally intensive tasks.
By exploring these advanced use cases, developers can unlock new possibilities and enhance the functionality of their applications using "foreach".
How is JavaScript foreach Used in Modern Web Development?
In modern web development, the "javascript foreach" method plays a crucial role in simplifying data manipulation and enhancing the efficiency of front-end and back-end applications. Its widespread adoption is driven by its ability to streamline code and support the functional programming paradigm, which is increasingly popular in contemporary software development.
Front-end frameworks like React, Angular, and Vue often utilize "foreach" to manage and render lists of components, enabling developers to efficiently update the DOM in response to data changes. By abstracting the iteration process, "foreach" allows developers to focus on building dynamic and interactive user interfaces without the overhead of manually managing loop counters.
On the back-end, "foreach" is frequently used in Node.js applications to process data streams, manage asynchronous workflows, and interact with databases. Its ability to handle large datasets efficiently makes it a valuable tool for back-end developers seeking to build scalable and performant applications.
Overall, "foreach" is an indispensable tool in modern web development, providing developers with the flexibility and power needed to build robust and maintainable applications.
How is JavaScript foreach Integrated into Frameworks and Libraries?
Many popular JavaScript frameworks and libraries integrate the "foreach" method as a core component, leveraging its simplicity and efficiency to streamline data manipulation and enhance code readability. These integrations often extend the functionality of "foreach" to suit the specific needs of the framework or library, providing developers with powerful tools for building complex applications.
In React, for example, "foreach" is commonly used to iterate over lists of components, allowing developers to dynamically render content based on data changes. By encapsulating the iteration logic within the component lifecycle, React developers can build highly responsive and interactive user interfaces with minimal code.
Similarly, libraries like Lodash and Underscore provide enhanced versions of "foreach" that offer additional features and optimizations, enabling developers to perform complex data manipulations with ease. These libraries often include utility functions that complement "foreach", such as "filter", "reduce", and "map", providing a comprehensive toolkit for functional programming.
By understanding how "foreach" is integrated into frameworks and libraries, developers can take full advantage of its capabilities and build more efficient and maintainable applications.
Are There Performance Considerations with JavaScript foreach?
When using "javascript foreach", it's essential to consider the potential performance implications, particularly when working with large datasets or computationally intensive tasks. While "foreach" is generally efficient for most applications, developers should be aware of its limitations and explore alternative methods when necessary.
One common performance concern is the overhead associated with function calls. Since "foreach" executes a callback function for each element of the array, the cumulative cost of these calls can become significant when processing large datasets. In such cases, developers may consider using traditional "for" loops or other optimized iteration methods to reduce the impact of function call overhead.
Another consideration is the potential for memory consumption. "foreach" does not create a new array, which can be beneficial in terms of memory efficiency. However, if the callback function involves creating new objects or data structures, developers should be mindful of the potential memory footprint and optimize their code accordingly.
By understanding these performance considerations and implementing best practices, developers can ensure that their applications remain efficient and responsive, even when using "foreach" with large datasets.
What Are the Security Implications of JavaScript foreach?
While "javascript foreach" is generally considered safe to use, developers should remain vigilant about potential security risks associated with its use. Ensuring secure code practices can help mitigate these risks and protect applications from vulnerabilities.
- Input Validation: Always validate and sanitize input data before processing it with "foreach". This practice helps prevent injection attacks and other security vulnerabilities by ensuring that data is clean and safe to use.
- Exception Handling: Implement robust exception handling within callback functions to manage potential errors and prevent them from propagating through the application. Proper error handling can help protect applications from crashing and maintain their stability.
- Access Control: Ensure that sensitive data is adequately protected during "foreach" operations. Implementing access control measures can help prevent unauthorized access to data and maintain the confidentiality of sensitive information.
By following these security best practices, developers can ensure that their use of "foreach" remains secure and does not expose their applications to unnecessary risks.
What Does the Future Hold for JavaScript foreach?
As JavaScript continues to evolve, the "foreach" method is expected to remain a fundamental tool for developers, with potential enhancements and optimizations on the horizon. As the language grows and adapts to new programming paradigms and technologies, "foreach" will likely continue to play a crucial role in simplifying data manipulation and iteration processes.
One potential area of development is the integration of native support for asynchronous operations within "foreach". Asynchronous workflows are becoming increasingly common in modern applications, and enhancing "foreach" to handle them natively could significantly simplify code and improve developer productivity.
Additionally, as JavaScript embraces new language features and standards, "foreach" may see further optimizations and enhancements to improve its performance and efficiency. These improvements could include better support for parallel processing, reduced function call overhead, and enhanced memory management capabilities.
Overall, the future of "foreach" is promising, with ongoing developments and innovations poised to enhance its functionality and maintain its status as an essential tool for JavaScript developers.
FAQs
- What is the difference between "foreach" and "map" in JavaScript?
"foreach" executes a function on each array element without returning a new array, while "map" returns a new array with the results of the function applied to each element.
- Can "foreach" be used with asynchronous operations?
While "foreach" is not inherently asynchronous, developers can use promises and async/await syntax to manage asynchronous workflows in conjunction with "foreach".
- Does "foreach" work with non-array objects?
No, "foreach" is specifically designed for array instances. Attempting to use it on non-array objects will result in a TypeError.
- How does "foreach" handle empty array elements?
"foreach" skips empty elements and does not execute the callback function for them, unlike methods like "map" or "reduce".
- Is "foreach" faster than a traditional "for" loop?
In most cases, the performance difference is negligible, but "for" loops can be faster for large datasets or when loop control variables are critical.
- What are some best practices for using "foreach"?
Use descriptive callback function names, keep functions concise, and minimize side effects to enhance code readability and maintainability.
Conclusion
The "javascript foreach" method is an indispensable tool for developers working with arrays in JavaScript. Its simplicity, efficiency, and alignment with functional programming principles make it a valuable asset for both front-end and back-end applications. By understanding how to use "foreach" effectively, developers can write cleaner, more maintainable code and enhance their ability to manipulate data in modern web development.
Throughout this article, we've explored the various aspects of "foreach", including its syntax, use cases, and best practices. We've also compared it to other iteration methods and discussed advanced applications and performance considerations. By following the guidelines and recommendations outlined here, developers can harness the full potential of "foreach" and build robust, efficient applications.
As JavaScript continues to evolve, "foreach" will remain a fundamental tool for developers, with ongoing innovations and enhancements poised to further improve its functionality and performance. By staying informed about these developments and adapting to new programming paradigms, developers can continue to leverage "foreach" to its fullest potential.
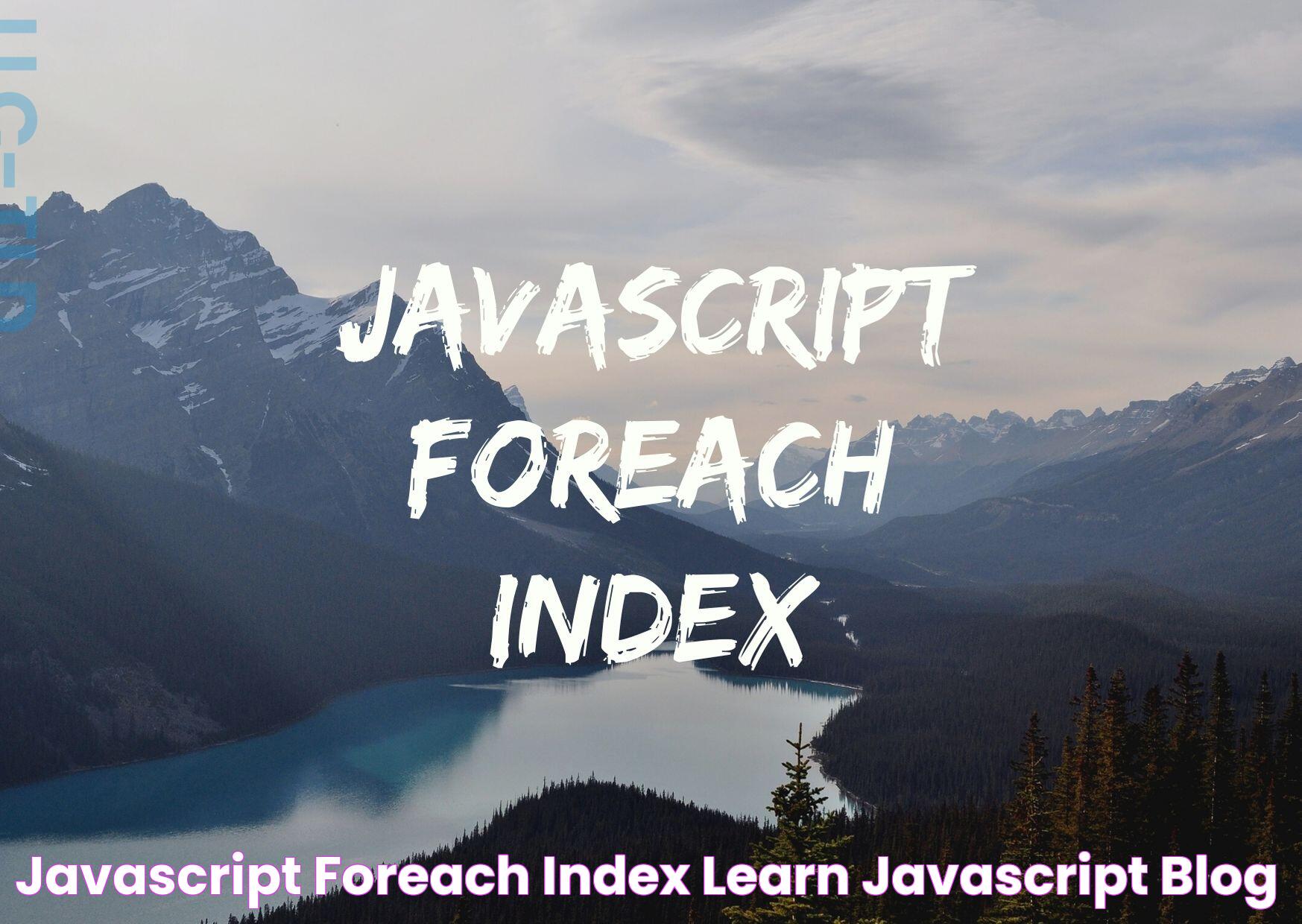
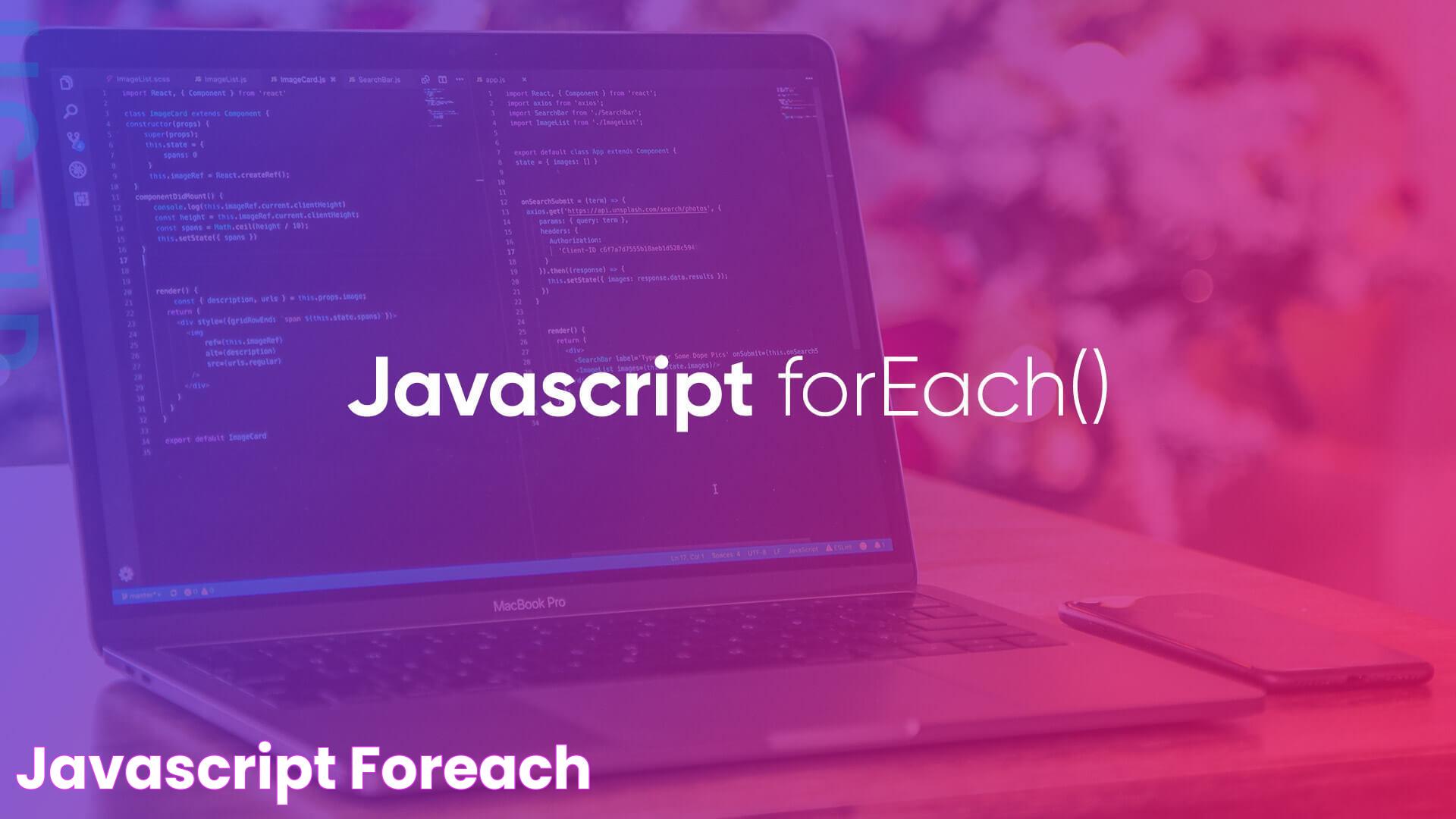