C# is part of the .NET framework and is widely utilized in creating desktop applications, web services, and even games using the Unity game engine. Its syntax is similar to other C-style languages like C++ and Java, making it easier for developers familiar with those languages to transition to C#. C#’s design also incorporates strong typing, lexically scoped, imperative, declarative, functional, generic, object-oriented, and component-oriented programming disciplines, making it a robust tool for developing a wide range of applications.
In this comprehensive guide, we’ll delve deep into the intricacies of the C Sharp Language. We’ll explore its history, fundamental concepts, advanced features, and real-world applications. Whether you’re aiming to build a simple application or a complex enterprise-level solution, this article will equip you with the knowledge and tools necessary to master C# and leverage its full potential in your projects.
Table of Contents
- What is the History of C Sharp Language?
- Understanding the Basics of C Sharp Language
- How Does C Sharp Syntax Work?
- Exploring Object-Oriented Programming in C#
- Advanced Features of C Sharp Language
- What Are the Essential Libraries and Frameworks in C#?
- Real-World Applications of C Sharp Language
- Common Errors and Debugging in C Sharp
- How to Optimize Your C Sharp Code?
- Security Considerations in C Sharp Programming
- What Tools and IDEs Are Recommended for C Sharp Development?
- Best Practices for Writing C Sharp Code
- The Future of C Sharp Language
- Frequently Asked Questions About C Sharp Language
- Conclusion
What is the History of C Sharp Language?
The journey of C Sharp Language began in the late 1990s when Microsoft set out to develop a language that would complement its .NET framework. Anders Hejlsberg, a prominent figure in the world of programming, spearheaded the development of C#, which was officially unveiled in 2000. C# was designed as part of the .NET initiative and was intended to be modern, simple, and object-oriented.
Read also:Efficiently Finding Products Look Up Item By Upc At Walmart
The introduction of C# marked a significant milestone in Microsoft's history as it aimed to compete with Java, a language that was gaining traction in the industry at the time. C# was built from the ground up to address some of the limitations of existing languages, offering better support for component-based programming and a more simplified syntax. Over the years, the language has evolved significantly, with Microsoft releasing regular updates that have introduced new features and improvements.
Today, C# is one of the most widely used programming languages, supported by a vast community of developers and an extensive ecosystem of tools and libraries. Its success can be attributed to its adaptability, ease of use, and the continuous support and innovation from Microsoft.
Understanding the Basics of C Sharp Language
Before diving into the complexities of C#, it's essential to grasp the fundamental concepts that form the foundation of the language. At its core, C# is an object-oriented programming language, which means it relies on classes and objects to organize code. Understanding these concepts is crucial for anyone looking to master C#.
Here are some of the basic building blocks of C#:
- Variables: Used to store data that can be changed during program execution. C# supports various data types, including integers, floats, strings, and more.
- Operators: Symbols used to perform operations on variables and values. C# has arithmetic, comparison, logical, and assignment operators.
- Control Structures: Constructs that control the flow of a program, such as loops (for, while) and conditional statements (if, switch).
- Methods: Blocks of code designed to perform specific tasks. Methods help in organizing code into reusable components.
- Classes and Objects: Classes are blueprints for creating objects. Objects are instances of classes that encapsulate data and behavior.
These basic concepts are integral to writing functional programs in C#. As you progress, you’ll learn how to combine these elements to create sophisticated applications.
How Does C Sharp Syntax Work?
C# syntax is designed to be clean, readable, and similar to other C-style languages, making it easier for developers to learn and transition from languages like C++ and Java. The syntax rules dictate how code is written and organized, ensuring that the compiler can understand and execute it correctly.
Read also:Stay Focused Quotes The Power Of Concentration And Determination
In C#, code is typically organized into namespaces, classes, and methods. A namespace is a container for classes and other types, helping to organize code and avoid naming conflicts. Classes define the structure of objects, while methods contain the logic that defines how a program behaves.
Here's a simple example of C# syntax:
namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } } }
In this example, the code is organized into a namespace called HelloWorld
, which contains a class named Program
. The Main
method is the entry point of the application, where execution begins. The Console.WriteLine
statement outputs the text "Hello, World!" to the console.
Understanding C# syntax is crucial for writing clear and efficient code. As you become more familiar with the rules and conventions, you'll be able to write complex programs with ease.
Exploring Object-Oriented Programming in C#
Object-oriented programming (OOP) is a cornerstone of C# and plays a significant role in its design and functionality. OOP is a programming paradigm that uses objects to represent data and behavior, making it easier to model real-world entities and relationships.
In C#, OOP principles include:
- Encapsulation: The practice of bundling data and methods that operate on that data within a single unit or class. This helps protect data from outside interference and misuse.
- Inheritance: The mechanism by which one class can inherit properties and methods from another class, promoting code reuse and reducing redundancy.
- Polymorphism: The ability to present the same interface for different data types, allowing for flexible and interchangeable code components.
- Abstraction: The concept of hiding complex implementation details and exposing only the necessary features of an object or class, simplifying interaction with the code.
By leveraging these principles, C# developers can create modular and maintainable code that closely mirrors real-world scenarios. OOP in C# allows for better organization, scalability, and collaboration on large projects.
Advanced Features of C Sharp Language
C# is not just about the basics; it offers a plethora of advanced features that empower developers to build complex and efficient applications. These features enhance productivity, improve code quality, and facilitate the development of robust solutions.
Some notable advanced features of C# include:
- LINQ (Language Integrated Query): A powerful querying capability integrated into C#, allowing developers to perform data manipulation and retrieval directly within the language.
- Asynchronous Programming: Using the
async
andawait
keywords, C# supports non-blocking operations, enabling the creation of responsive applications that handle concurrent tasks efficiently. - Delegates and Events: Delegates are type-safe function pointers that enable callbacks and event handling, while events provide a way to notify subscribers when something of interest occurs.
- Generics: A feature that allows developers to define classes, methods, and interfaces with placeholder types, promoting code reusability and type safety.
- Reflection: The ability to inspect and interact with objects and assemblies at runtime, facilitating dynamic programming and advanced debugging techniques.
These advanced features make C# a versatile language capable of handling a wide range of programming tasks, from developing simple applications to creating complex enterprise solutions.
What Are the Essential Libraries and Frameworks in C#?
C# is part of the .NET ecosystem, which provides a rich set of libraries and frameworks that extend the language's capabilities and simplify development tasks. These libraries and frameworks offer pre-built functionality that developers can leverage to streamline their workflows and enhance their applications.
Some essential libraries and frameworks in C# include:
- .NET Framework: A comprehensive and consistent programming model for building applications with visually stunning user experiences and seamless communication.
- .NET Core: A cross-platform framework for developing applications that can run on Windows, macOS, and Linux, offering improved performance and scalability.
- ASP.NET: A framework for building web applications and services, providing robust tools for creating dynamic and interactive websites.
- Entity Framework: An object-relational mapper (ORM) that enables developers to work with databases using .NET objects, simplifying data access and manipulation.
- Xamarin: A framework for building cross-platform mobile applications using C#, allowing code sharing across iOS, Android, and Windows platforms.
By utilizing these libraries and frameworks, C# developers can accelerate the development process, reduce complexity, and deliver high-quality applications across various platforms and environments.
Real-World Applications of C Sharp Language
C# is a versatile language with a wide range of applications across different industries and domains. Its flexibility, performance, and ease of use make it an ideal choice for developing various types of software solutions.
Here are some real-world applications of C#:
- Windows Applications: C# is commonly used for creating Windows desktop applications, thanks to its seamless integration with the Windows operating system and the .NET framework.
- Web Development: With ASP.NET, C# developers can build dynamic and scalable web applications and services, catering to various business needs.
- Game Development: C# is the primary language used in Unity, a popular game engine, enabling developers to create 2D and 3D games for multiple platforms.
- Mobile Applications: Using Xamarin, C# developers can create cross-platform mobile apps that run on iOS, Android, and Windows devices, ensuring a consistent user experience.
- Enterprise Software: C# is widely used in developing enterprise-level applications, such as customer relationship management (CRM) systems and enterprise resource planning (ERP) solutions.
The diverse applications of C# demonstrate its versatility and effectiveness in addressing a wide range of programming challenges. Whether you're developing desktop software, web applications, or mobile games, C# provides the tools and features needed to succeed.
Common Errors and Debugging in C Sharp
Even the most experienced developers encounter errors in their code, and C# is no exception. Understanding common errors and how to debug them is essential for maintaining the integrity and functionality of your applications.
Here are some common errors in C# and tips for debugging:
- Syntax Errors: These occur when the code does not conform to the language's syntax rules. Use a code editor with syntax highlighting to identify and correct these errors.
- NullReferenceException: This error occurs when you attempt to access a member on a null object. Use null checks and the
?.
operator to prevent this error. - IndexOutOfRangeException: Occurs when trying to access an array or collection element that does not exist. Always validate index values before accessing elements.
- Type Mismatch: This error occurs when attempting to assign a value of one type to a variable of another incompatible type. Use type casting or conversion methods to resolve this error.
Debugging is an essential skill for any developer. Use debugging tools available in your IDE, such as breakpoints and watch windows, to step through your code and identify the root cause of errors. By understanding common errors and employing effective debugging techniques, you can ensure the reliability and stability of your C# applications.
How to Optimize Your C Sharp Code?
Optimizing your C# code is crucial for improving performance, reducing resource consumption, and ensuring a smooth user experience. Various techniques can be employed to achieve these goals, from refining algorithms to utilizing efficient data structures.
Here are some strategies for optimizing C# code:
- Efficient Data Structures: Choose appropriate data structures based on the problem requirements, such as using
Dictionary
for quick lookups instead ofList
. - Minimize Memory Usage: Avoid unnecessary object creation and use value types instead of reference types where applicable to reduce memory overhead.
- Optimize Loops: Minimize the number of iterations and calculations within loops. Consider using parallel processing for tasks that can be executed concurrently.
- Use Asynchronous Programming: Employ async and await to perform non-blocking operations, improving the responsiveness of applications.
- Profile and Analyze: Use profiling tools to identify performance bottlenecks and optimize code based on data-driven insights.
By implementing these optimization techniques, you can enhance the performance and efficiency of your C# applications, delivering a better experience to your users.
Security Considerations in C Sharp Programming
Security is a critical aspect of software development, and C# provides various features and best practices to help developers build secure applications. Addressing security concerns early in the development process can prevent vulnerabilities and protect sensitive data.
Here are some security considerations for C# programming:
- Input Validation: Always validate and sanitize user input to prevent injection attacks and ensure data integrity.
- Secure Data Storage: Use encryption to protect sensitive data at rest and in transit, preventing unauthorized access.
- Access Control: Implement role-based access control to restrict access to sensitive features and data based on user permissions.
- Exception Handling: Properly handle exceptions to avoid exposing sensitive information through error messages.
- Regular Updates: Keep your development environment, libraries, and frameworks up to date to mitigate known vulnerabilities.
By incorporating these security considerations into your C# applications, you can safeguard your software from potential threats and ensure the confidentiality, integrity, and availability of your data.
What Tools and IDEs Are Recommended for C Sharp Development?
Choosing the right tools and integrated development environments (IDEs) can significantly enhance your productivity and streamline the C# development process. These tools provide essential features such as code editing, debugging, and project management, making development more efficient and enjoyable.
Here are some recommended tools and IDEs for C# development:
- Visual Studio: A comprehensive IDE from Microsoft, offering advanced features such as IntelliSense, debugging, and version control integration, making it the preferred choice for C# developers.
- Visual Studio Code: A lightweight and versatile code editor with support for C# through extensions, suitable for developers seeking a customizable and cross-platform solution.
- Rider: An IDE by JetBrains that provides a rich set of features, including code analysis, refactoring, and debugging, tailored for C# and .NET development.
- LINQPad: A tool for testing and prototyping C# code snippets, offering a convenient environment for experimenting with LINQ queries and other C# features.
- ReSharper: A Visual Studio extension that enhances code quality and productivity with powerful code analysis, refactoring, and navigation capabilities.
By leveraging these tools and IDEs, C# developers can streamline their workflows, improve code quality, and deliver high-quality applications more efficiently.
Best Practices for Writing C Sharp Code
Adhering to best practices is essential for writing clean, maintainable, and efficient C# code. These practices help ensure consistency, reduce errors, and improve collaboration among developers working on the same project.
Here are some best practices for writing C# code:
- Consistent Naming Conventions: Use meaningful and descriptive names for variables, methods, and classes, following standard naming conventions such as camelCase for variables and PascalCase for class names.
- Code Readability: Write code that is easy to read and understand, using proper indentation, spacing, and comments to explain complex logic.
- DRY Principle: Follow the "Don't Repeat Yourself" principle by avoiding code duplication and using reusable methods and classes.
- Unit Testing: Write unit tests to verify the correctness of your code and ensure that changes do not introduce new bugs.
- Continuous Integration: Implement continuous integration practices to automate testing and build processes, promoting faster and more reliable releases.
By incorporating these best practices into your C# development workflow, you can create high-quality code that is easier to understand, maintain, and extend.
The Future of C Sharp Language
The future of C# is promising, with Microsoft and the developer community continuously investing in its growth and evolution. As technology advances, C# is expected to adapt and incorporate new features and improvements that align with emerging trends and needs.
Some potential developments in the future of C# include:
- Cross-Platform Compatibility: Continued efforts to enhance cross-platform capabilities, making it easier to develop applications that run seamlessly on various operating systems.
- Cloud Integration: Increased focus on cloud-based development, with tools and frameworks that facilitate the creation of scalable and distributed applications.
- AI and Machine Learning: Integration of artificial intelligence and machine learning capabilities, empowering developers to build intelligent and data-driven applications.
- Enhanced Language Features: Ongoing introduction of new language features and improvements that simplify development and enhance performance.
With its strong foundation and continuous innovation, C# is poised to remain a leading language in the programming world, offering developers the tools and capabilities needed to tackle future challenges and opportunities.
Frequently Asked Questions About C Sharp Language
1. What is C Sharp Language used for?
C# is a versatile programming language used for developing a wide range of applications, including Windows desktop applications, web services, mobile apps, and games. Its integration with the .NET framework and support for object-oriented programming make it suitable for various development tasks.
2. Is C Sharp Language easy to learn?
C# is considered relatively easy to learn, especially for developers familiar with other C-style languages like C++ and Java. Its clean syntax, extensive documentation, and supportive community make it accessible to beginners and experienced developers alike.
3. Can C Sharp Language be used for web development?
Yes, C# can be used for web development through the ASP.NET framework, which allows developers to create dynamic and scalable web applications and services. ASP.NET provides tools and libraries that simplify the development of web-based solutions.
4. How does C Sharp Language handle memory management?
C# uses automatic memory management through a process called garbage collection. The garbage collector automatically frees up memory by reclaiming unused objects, reducing the risk of memory leaks and simplifying memory management for developers.
5. What are the advantages of using C Sharp Language?
The advantages of using C# include its ease of use, strong typing, and support for object-oriented programming. Additionally, its integration with the .NET framework, extensive libraries, and cross-platform capabilities make it a powerful tool for developing diverse applications.
6. Is C Sharp Language suitable for game development?
Yes, C# is widely used in game development, particularly with the Unity game engine. Unity supports C# scripting, enabling developers to create interactive and immersive 2D and 3D games for multiple platforms, including PC, consoles, and mobile devices.
Conclusion
In conclusion, mastering the C Sharp Language opens up a world of opportunities in the realm of software development. Its versatility, ease of use, and integration with the .NET framework make it a powerful tool for building a wide range of applications, from desktop software to web services and games. By understanding its history, fundamental concepts, advanced features, and best practices, developers can leverage C# to create innovative and efficient solutions that meet the demands of today's technology-driven world.
As we look to the future, C# continues to evolve, offering new features and capabilities that empower developers to tackle emerging challenges and explore new possibilities. With a strong community, extensive resources, and continuous support from Microsoft, C# remains a valuable language for developers at all levels, enabling them to bring their ideas to life and make a meaningful impact in the tech industry.
Whether you're a beginner or an experienced developer, embracing the C Sharp Language can enhance your skill set, boost your career, and open doors to exciting opportunities in the ever-evolving world of programming.
For more information on C# and its applications, you can visit the official C# documentation provided by Microsoft.
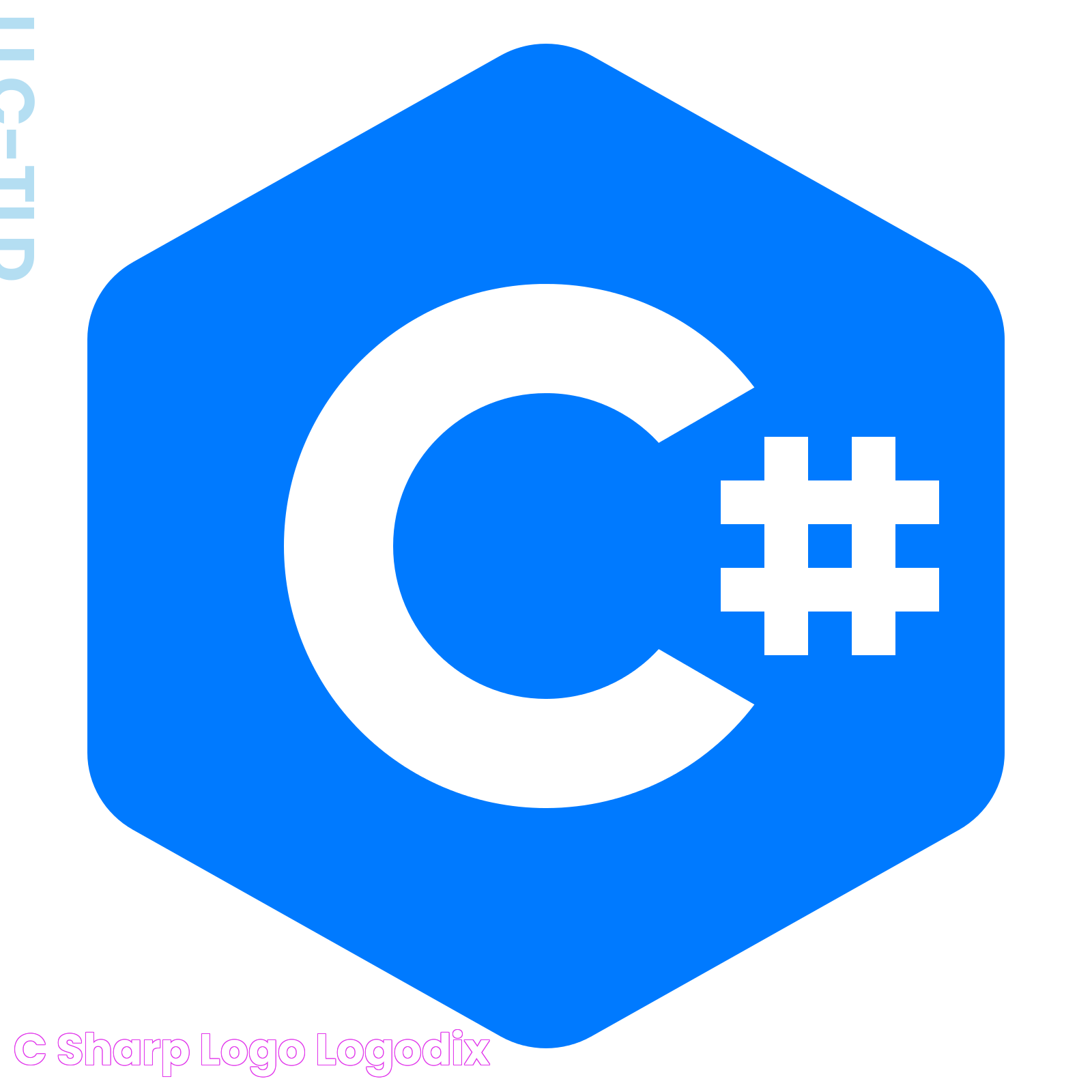
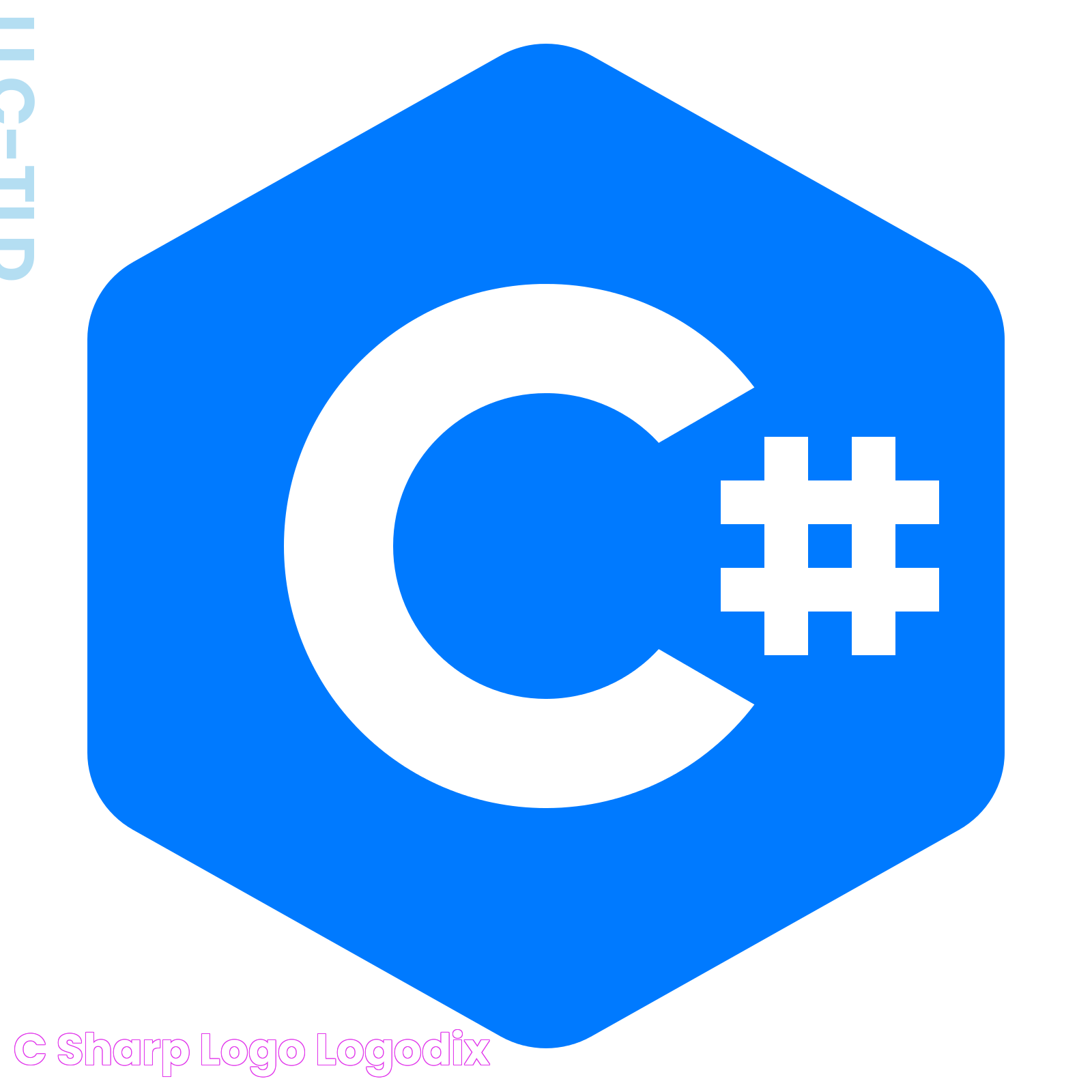